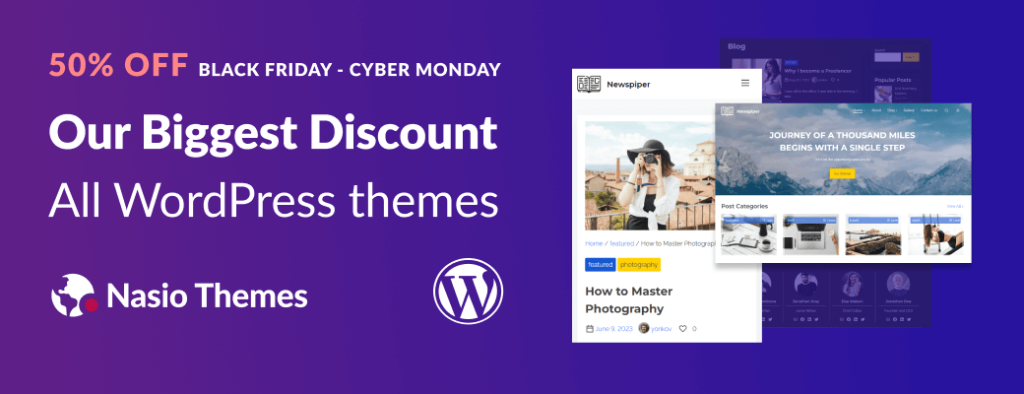
In this tutorial, I will show you how to easily schedule a repetitive task to automatically open websites from the browser in Ubuntu.
Cron is the Linux way to schedule tasks. If you are a Windows user, you probably know that in Windows OS you can easily add repeated processes via the Windows Task Scheduler user interface. The standard Unix/Linux/Ubuntu way of scheduling is using the cron
service. Cron is a time-based job scheduling daemon found in Unix-like operating systems, including Linux distributions.
How does Cron Work?
Cron jobs are recorded and managed in a special file known as a crontab
. Each user profile has their own crontab, where they can add all their scheduled tasks. Adding a cron job is as simple as opening up your crontab
for editing and adding a task written in the form of a cron expression. Each cron expression consists of two elements: the schedule and the command to run.
How to create a Cron Job?
Most distributions already have some kind of cron installed. To check if you have it, open the terminal (ctrl + alt + T ) and run the following command: man cron
. If you don’t have it installed, run the following commands to set it up:
sudo apt install cron sudo systemctl enable cron
To schedule a cron job, run
crontab -e
Why Ping a Website?
Pinging websites is a useful technique that has many valid use cases. For example, it can be used to monitor the uptime of a website, analyse response times, content updates, etc. Moreover, WordPress websites rely heavily on page loads as a way to trigger their own task scheduling and check for plugin and theme updates.
How to Ping a Website Once Per Day?
Pick your editor of choice and add the following code in the crontab:
0 11 * * * /bin/ping -c 1 www.google.com > /dev/null 2>&1
The above cron job will ping google via the command line every day from 11a.m. Let’s break down to code, so you have a better understanding what is going on:
0
: This is the minute field and means “on the 0th minute” (i.e., at the start of the hour).11
: This is the hour field and means “at 11 o’clock”.* * *
: These are the day of the month, month, and day of the week fields, respectively. The asterisks (*) indicate that the command will run every day of the month and every day of the week./bin/ping -c 1 www.example.com
: This is the command to be executed. It uses theping
command to send one packet to the websitewww.google.com
.> /dev/null 2>&1
: This part discards the standard output and standard error, so you won’t receive email notifications for the ping. If you want to see an error log for debugging reasons, change “/dev/null” part to “/tmp/cron.log
“
Opening Websites from the Browser
There are some situations when you would actually want not just to ping a website on a regular basis but to actually visit it. In such a situation, you can edit the above crone in the following way:
0 11 * * * DISPLAY=:0 /snap/bin/firefox https://www.google.com > /dev/null 2>&
If you are not sure about your browser path, you can check it with the which
command:
which firefox
While the above code works, what if we want to open more than one website?
0 11 * * * DISPLAY=:0 /snap/bin/firefox https://www.example.com https://www.google.com https://www.youtube.com >> /tmp/cron.log 2>&1
Bonus: Opening Websites with a Delay
This is nice but what if we want to go a step further. Let’s say we want to open the websites with a delay? For this, it will be better to source the logic into a seperate bash script and then call it from the cron job Here’s how you can do it:
1. Create a new file, for example open-websites.sh
2. Add the following code:
#!/bin/bash
# Open websites with delays
websites=(
"https://example.com"
"https://www.google.com"
"https://youtube.com"
)
for site in "${websites[@]}"
do
/snap/bin/firefox --new-tab "$site"
sleep 9
done
3. Make the file executable:
chmod +x open-websites.sh
Save and close the file (in Nano, you can do this by pressing Ctrl + X
, then Y
, then Enter
).
4. Adjust the cron job to run scripts
Finally, adjust the cron in the crontab to run the script we have just created:
0 11 * * * DISPLAY=:0 "/home/your_username/Downloads/scripts/open-websites.sh" >> /tmp/cron.log 2>&1
Don’t forget to change the path to the place where you have saved the script. Congratulations, you have learnt how to create simple but useful cron job in Ubuntu that will monitor your website on a daily basis!